When working with sensitive data in your application, it's important to protect it from unauthorized access. One key part of security is controlling how sensitive information appears in logs. In this article, we’ll explore how ReportPortal hides confidential data.
Logging helps developers and testers understand how an application works, find errors, and track tests. However, logs can sometimes include sensitive details like API keys, passwords, or access tokens. This can be a security risk, so it’s important to know how to prevent such data from being exposed. To demonstrate filtering capabilities, we will be using the REST Assured logger in ReportPortal of version 5.3.9, since this version contains all the necessary functionality.
ReportPortal ensures data security by:
Removing sensitive information from the "Authorization" header
Filtering out confidential data from headers, URLs, and cookies
Masking sensitive content in response bodies
Case #1: Removing sensitive information from the "Authorization" header
A while ago, the REST Assured logger in ReportPortal started supporting excluding sensitive information from blacklisted header configuration of REST Assured. You can do this by specific configuration of REST Assured: `RestAssuredConfig.config().logConfig(LogConfig.logConfig().blacklistHeader("Authorization"))`.
Here is a full example:
import com.epam.reportportal.listeners.*;
import com.epam.reportportal.restassured.ReportPortalRestAssuredLoggingFilter;
import com.epam.reportportal.service.Launch;
import io.restassured.RestAssured;
import io.restassured.config.*;
import org.testng.annotations.*;
import static java.util.Optional.ofNullable;
public class RestAssuredSimpleSanitizeTest {
private static final RestAssuredConfig CONFIG = RestAssuredConfig.config()
.logConfig(LogConfig.logConfig().blacklistHeader("Authorization"));
@BeforeClass
public void setupRestAssured() {
RestAssured.reset(); // Reset everything to avoid collisions with other REST Assured examples
RestAssured.filters(new ReportPortalRestAssuredLoggingFilter(42, LogLevel.INFO));
}
@Test
public void simpleRestAssuredLoggingTest() {
ListenerParameters parameters = ofNullable(Launch.currentLaunch()).map(Launch::getParameters)
.orElseThrow(() -> new IllegalStateException("Launch is not started"));
RestAssured.given()
.config(CONFIG)
.header("Authorization", "Bearer " + parameters.getApiKey())
.get(parameters.getBaseUrl() + "/api/v1/" + parameters.getProjectName() + "/settings")
.then()
.assertThat()
.statusCode(200);
}
}
Here is how it looks on ReportPortal:
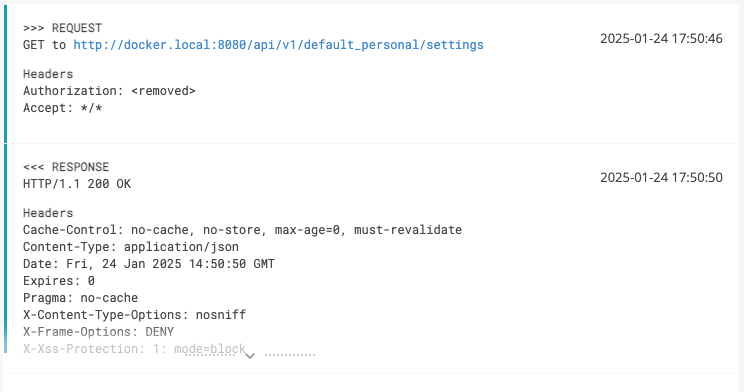
So we see that the "Authorization" header is hidden in the logs, while other headers and request/response bodies are logged as usual. It's a step in the right direction for securing sensitive information in logs, but it can't be the complete solution. Modern API use cases almost always involve more complex sensitive data transfer cases, which require more sophisticated handling of sensitive data. Fortunately, ReportPortal provides additional converters and prettifiers that help exclude such data from logs.
Case #2: Removing sensitive information from headers, URL, and cookies
Imagine you are testing a browser authorization mechanism, which involves HTTP request that includes sensitive information in the headers and URL, E.G. basic authorization header with client credentials and user credentials in the URL. And in response to the request server sets Cookies with the Session ID to authorize the browser, therefore Session ID should be also confidential. Let's see what we will have in logs in this case:
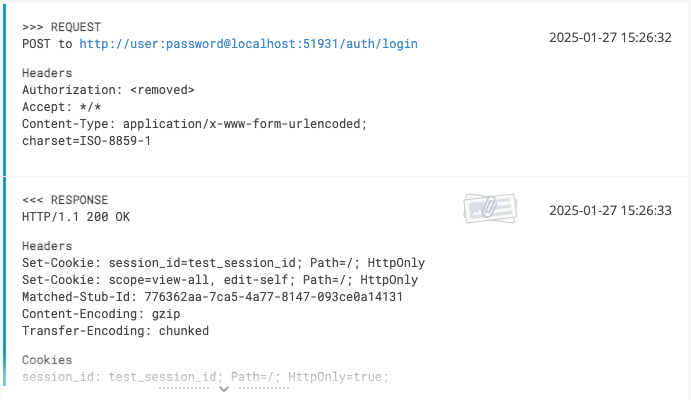
It doesn't look good, right? So, what can we do to hide this sensitive information? First, since all filers and converters are working both on request and response logs, we can use the same `blacklistHeader` method to hide "Set-Cookie" header in the response. But we still have to deal with URL credentials the request and separate Cookie logging in the response. For this purpose, ReportPortal provides `SanitizingUriConverter` converter, which removes passwords from URIs, and `SanitizingCookieConverter` converter, which removes Session IDs from cookies.
So our REST Assured configuration becomes like this:
private static final RestAssuredConfig CONFIG = RestAssuredConfig.config()
.logConfig(LogConfig.logConfig().blacklistHeader("Authorization").blacklistHeader("Set-Cookie"));
And filter configuration is also a bit different:
RestAssured.filters(new ReportPortalRestAssuredLoggingFilter(
42,
LogLevel.INFO,
DefaultHttpHeaderConverter.INSTANCE,
DefaultHttpHeaderConverter.INSTANCE,
SanitizingCookieConverter.INSTANCE,
SanitizingUriConverter.INSTANCE
));
Here is how it looks on ReportPortal now:
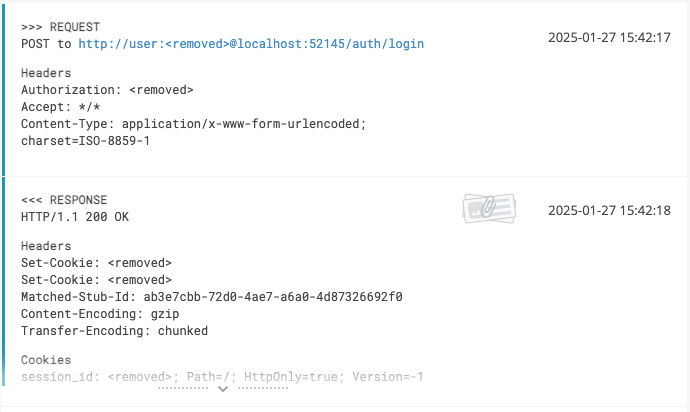
Way better!
Case #3: Removing sensitive information from response body
Let's look at another example, where we have a response body with sensitive information, which we want to hide from logs. For example, we are testing an oAuth 2.0 authorization flow, where we have a response body with an access token and refresh token. Here is how it looks like in logs:
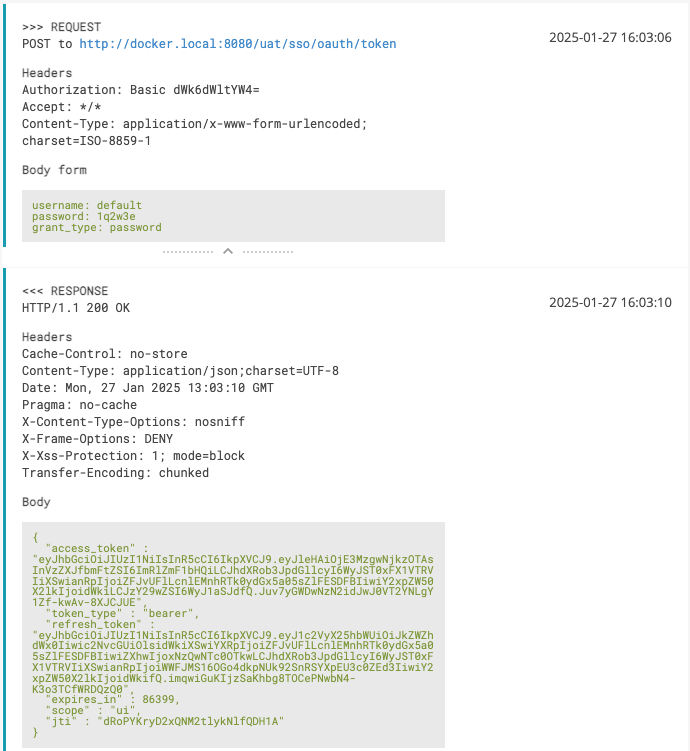
So here we see the following data which needs to be sanitized: Basic Authorization header, "password" form parameter, "access_token" and refresh_token" fields in the response body. To shorten the configuration, we will use `SanitizingHttpHeaderConverter` class from ReportPortal libraries, which hides the "Authorization" header.
Next we need to step aside a bit to explain what Converter is and what is Prettifier in ReportPortal. Converter is a class that converts an object (Header, URI, Param) to a string, which then is logged to ReportPortal. Prettifier is a class that formats a string for better readability. Naturally, HTTP request/response parts such as Header, URI, Param and Cookie require converters, while Body requires prettifier.
So, form parameters pass through `DefaultFormParamConverter` converter to be logged, and `ReportPortalRestAssuredLoggingFilter` class allows to configure custom converter for form parameters, with which we can hide "password" parameter. Let's create this custom converter:
private static final Function<Param, String> SANITIZING_PARAM_CONVERTER = new Function<>() {
@Override
public @Nullable String apply(@Nullable Param param) {
return DefaultFormParamConverter.INSTANCE.apply(ofNullable(param).filter(p -> "password".equalsIgnoreCase(p.getName()))
.map(p -> {
Param newParam = p.clone();
newParam.setValue("<removed>");
return newParam;
})
.orElse(param));
}
};
What we did here is we check if the parameter name is "password" (ignoring case) and if it is, we replace its value with "<removed>". Otherwise, we just return the parameter as is. We also then apply `DefaultFormParamConverter` to convert the resulting parameter to a string.
With prettifiers it's a bit harder to configure, since `ReportPortalRestAssuredLoggingFilter` class has its own prettifier for every body type (JSON, XML, Text, etc.) and the class does not allow modifying them on the fly. So, we need to get all of them, create our own prettifier, which will use the original prettifier to format the body and replace the sensitive information with "<removed>" tag and then replace the original prettifier with our own. Here is how it looks like:
@NotNull
private static Map<String, Function<String, String>> getUpdatedPrettifiersMap(@Nonnull Map<String, Function<String, String>> prettifiers) {
Map<String, Function<String, String>> myPrettifiers = new HashMap<>(prettifiers);
Function<String, String> jsonPrettifier = myPrettifiers.get("application/json");
Function<String, String> jsonSanitizer = json -> jsonPrettifier.apply(json.replaceAll(
"access_token\"(\\s*):(\\s*)\"[^\"]*\"",
"access_token\"$1:$2\"<removed>\""
)
.replaceAll("refresh_token\"(\\s*):(\\s*)\"[^\"]*\"", "refresh_token\"$1:$2\"<removed>\""));
myPrettifiers.put("application/json", jsonSanitizer);
return myPrettifiers;
}
@BeforeClass
public void setupRestAssured() {
RestAssured.reset(); // Reset everything to avoid collisions with other REST Assured examples
ReportPortalRestAssuredLoggingFilter logger = new ReportPortalRestAssuredLoggingFilter(
42,
LogLevel.INFO,
SanitizingHttpHeaderConverter.INSTANCE,
DefaultHttpHeaderConverter.INSTANCE,
DefaultCookieConverter.INSTANCE,
DefaultUriConverter.INSTANCE,
SANITIZING_PARAM_CONVERTER
);
logger.setContentPrettifiers(getUpdatedPrettifiersMap(logger.getContentPrettifiers()));
RestAssured.filters(logger);
}
And here is how it looks like on ReportPortal: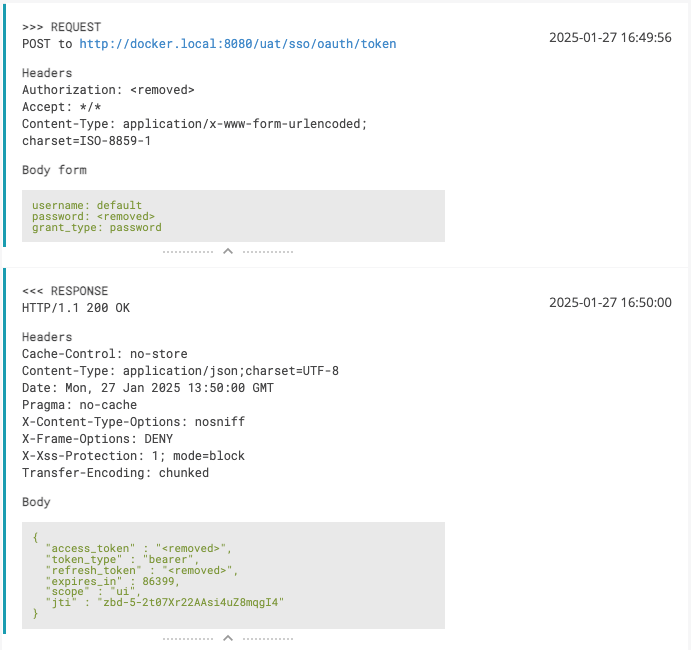
Cool! Now we have covered all the cases of sensitive information exposure in logs. And to recap, here is the full test class:
package com.epam.reportportal.example.testng.logback.logging.restassured;
import com.epam.reportportal.formatting.http.converters.*;
import com.epam.reportportal.formatting.http.entities.Param;
import com.epam.reportportal.listeners.*;
import com.epam.reportportal.restassured.ReportPortalRestAssuredLoggingFilter;
import com.epam.reportportal.service.Launch;
import io.restassured.RestAssured;
import org.testng.annotations.*;
import javax.annotation.*;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
import java.util.HashMap;
import java.util.Map;
import java.util.function.Function;
import static java.util.Optional.ofNullable;
public class RestAssuredFormTest {
private static final Function<Param, String> SANITIZING_PARAM_CONVERTER = new Function<>() {
@Override
public @Nullable String apply(@Nullable Param param) {
return DefaultFormParamConverter.INSTANCE.apply(ofNullable(param).filter(p -> "password".equalsIgnoreCase(p.getName()))
.map(p -> {
Param newParam = p.clone();
newParam.setValue("<removed>");
return newParam;
})
.orElse(param));
}
};
@Nonnull
private static Map<String, Function<String, String>> getUpdatedPrettifiersMap(@Nonnull Map<String, Function<String, String>> prettifiers) {
Map<String, Function<String, String>> myPrettifiers = new HashMap<>(prettifiers);
Function<String, String> jsonPrettifier = myPrettifiers.get("application/json");
Function<String, String> jsonSanitizer = json -> jsonPrettifier.apply(json.replaceAll(
"access_token\"(\\s*):(\\s*)\"[^\"]*\"",
"access_token\"$1:$2\"<removed>\""
)
.replaceAll("refresh_token\"(\\s*):(\\s*)\"[^\"]*\"", "refresh_token\"$1:$2\"<removed>\""));
myPrettifiers.put("application/json", jsonSanitizer);
return myPrettifiers;
}
@BeforeClass
public void setupRestAssured() {
RestAssured.reset(); // Reset everything to avoid collisions with other REST Assured examples
ReportPortalRestAssuredLoggingFilter logger = new ReportPortalRestAssuredLoggingFilter(
42,
LogLevel.INFO,
SanitizingHttpHeaderConverter.INSTANCE,
DefaultHttpHeaderConverter.INSTANCE,
DefaultCookieConverter.INSTANCE,
DefaultUriConverter.INSTANCE,
SANITIZING_PARAM_CONVERTER
);
logger.setContentPrettifiers(getUpdatedPrettifiersMap(logger.getContentPrettifiers()));
RestAssured.filters(logger);
}
@Test
public void restAssuredLoggingTest() {
ListenerParameters parameters = ofNullable(Launch.currentLaunch()).map(Launch::getParameters)
.orElseThrow(() -> new IllegalStateException("Launch is not started"));
RestAssured.given()
.header("Authorization", "Basic " + Base64.getEncoder().encodeToString("ui:uiman".getBytes(StandardCharsets.UTF_8)))
.formParam("username", "default")
.formParam("password", "1q2w3e")
.formParam("grant_type", "password")
.post(parameters.getBaseUrl() + "/uat/sso/oauth/token")
.then()
.assertThat()
.statusCode(200);
}
}
In this article, we explored how to protect sensitive information in logs when using ReportPortal and REST Assured logger. We covered three cases of sensitive information exposure in logs and how to handle them using ReportPortal's built-in functionality. `ReportPortalRestAssuredLoggingFilter` class has converters and prettifiers configuration which we can use and extend to cover our needs. We also learned how to create custom converters and prettifiers to handle specific cases of sensitive information exposure in logs.